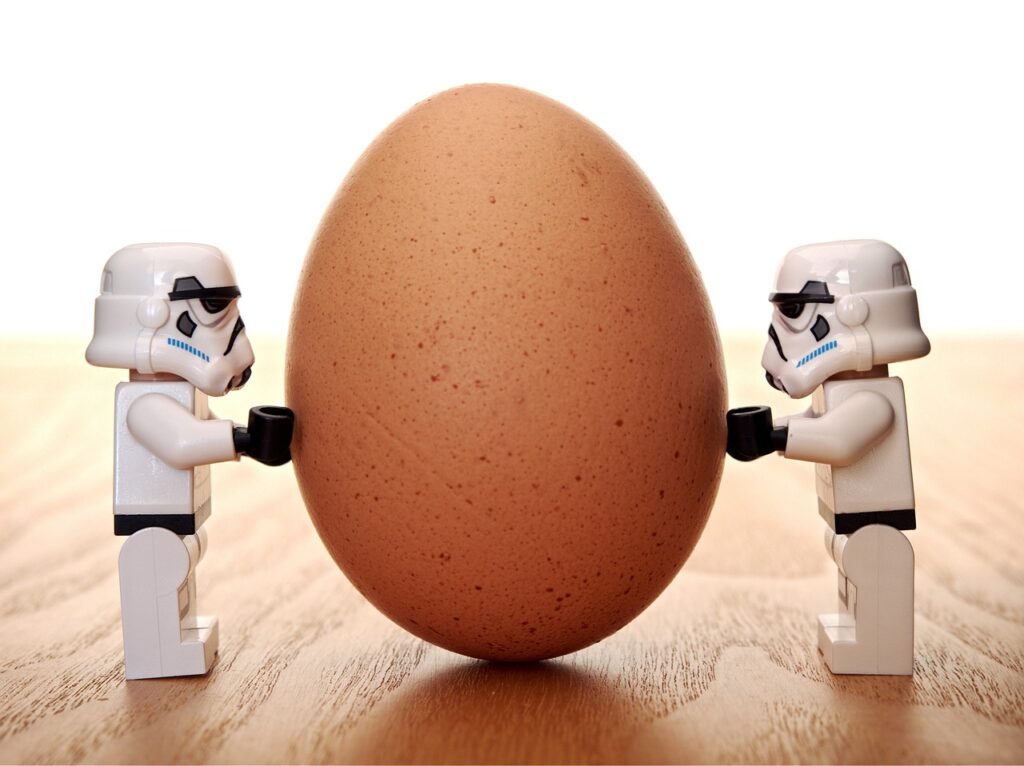
Context
Unit testing plays a crucial role in modern software development, ensuring the reliability and quality of code. When it comes to unit testing in Kotlin, Mockk is a popular framework that offers powerful features for mocking and stubbing dependencies. In this blog post, we will explore the fundamentals of Mockk and provide real-world examples to help you master this incredible tool.
Getting Started with Mockk
To start using Mockk, you need to add the necessary dependencies to your Kotlin project. Mockk is available on Maven Central, so you can easily include it in your build.gradle file:
dependencies { testImplementation("io.mockk:mockk:1.13.8") }
Once you have added the dependency, you’re ready to dive into the Mockk functionalities.
Basic Mocking and Stubbing
First, let’s take a look at how to create a mock object using Mockk. Imagine a simple UserService interface with a getUsername
method that returns a username:
interface UserService {
fun getUsername(): String
}
To create a mock object of this interface, use the mockk
function:
val userServiceMock = mockk<UserService>()
Now, you can define the behavior of the mocked object using Mockk’s every
function. Let’s stub the getUsername
method to return a static value:
every { userServiceMock.getUsername() } returns "JohnDoe"
Here, we are instructing the mock object to return “JohnDoe” whenever the getUsername
method is invoked.
Verifying Calls
Mockk allows you to verify if certain methods have been called on a mock object. Consider the following example:
val userServiceMock = mockk<UserService>()
userServiceMock.getUsername()
verify { userServiceMock.getUsername() }
In this case, we are verifying that the getUsername
method is called on the mock object.
Capturing Arguments
Mockk allows you to capture arguments passed to mocked methods using its slot
feature. Let’s see an example:
val userServiceMock = mockk<UserService>()
val capturedUsername = slot<String>()
every { userServiceMock.setUsername(capture(capturedUsername)) } just Runs
userServiceMock.setUsername("JohnDoe")
assertThat(capturedUsername.captured).isEqualTo("JohnDoe")
In this example, we are capturing the argument passed to the setUsername
method and asserting its value.
Mocking Final Classes and Objects
Mockk is also capable of mocking final classes and objects, which is not possible with some other mocking frameworks. Here’s an example of mocking a final class:
final class FinalClass {
fun finalMethod(): Int {
return 42
}
}
val finalClassMock = mockk<FinalClass>()
every { finalClassMock.finalMethod() } returns 24
assertThat(finalClassMock.finalMethod()).isEqualTo(24)
Using Mockk in JUnit Tests
To initialize mocks in JUnit5 tests, you need to annotate your test class with @ExtendWith(MockKExtension::class)
from the Mockk library.
Here’s an example:
@ExtendWith(MockKExtension::class)
class CarTest {
@MockK
lateinit var car1: Car
@RelaxedMockK
lateinit var car2: Car
@MockK(relaxUnitFun = true)
lateinit var car3: Car
@SpyK
var car4 = Car()
@Test
fun calculateAddsValues1() {
// ... use car1, car2, car3 and car4
}
}
Now, you can use Mockk’s mocking and verifying functionalities within your test methods.
Conclusion
Mockk is a powerful tool for unit testing in Kotlin, providing features for mocking, stubbing, and verifying method calls. This blog post has covered the basics of Mockk and provided practical examples to help you get started. With Mockk, you can easily write reliable and efficient unit tests, leading to better software quality and faster development cycles.
Remember to refer to the official Mockk documentation for more advanced features and specific use cases. Happy mocking!
In the world of unit testing, creating mock objects is essential for isolating dependencies and testing specific components in isolation. One popular tool that has gained a lot of attention in recent years is Mockk. This powerful mocking library for Kotlin provides developers with a seamless way to write expressive and concise tests.